HttpHandler and how to create your own http handler :
1. Create a class that implements IHttpHandler interface
2. Expose properties and method IsReusable and ProcessRequest
Step 1: File ---> New Project ---> ASP .Net Web Application
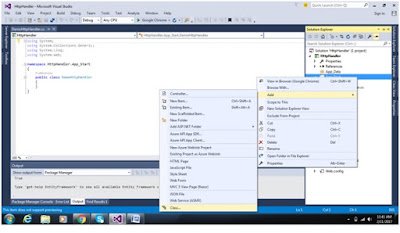
2: Create Class DemoHttpHandler and Implement IHttpHandler
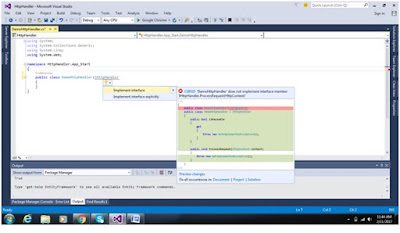
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Routing;
namespace HttpHandler.App_Start
{
public class DemoHttpHandler : IHttpHandler
{
public RequestContext RequestContext
{
get;
set;
}
public DemoHttpHandler(RequestContext reqCon)
{
RequestContext = reqCon;
}
public bool IsReusable
{
get
{
return true;
}
}
public void ProcessRequest(HttpContext context)
{
context.Response.Write("Hello Sandeep");
}
}
}
3: We need Route Handler the you create another class with
name RouteHandler show below screen
Route Handler implement using the using System.Web.Routing;
Namespace and implement IRouteHandler shows below code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Routing;
namespace HttpHandler.App_Start
{
public class RouteHandler : IRouteHandler
{
public IHttpHandler GetHttpHandler(RequestContext requestContext)
{
//call
Parameterised constructor in DemoHttpHandler
return new DemoHttpHandler(requestContext);
}
}
}
4: Register your HttpHandler in the RouteConfig File
using HttpHandler.App_Start;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Routing;
namespace HttpHandler
{
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.Add(new Route("Home/Demo", new RouteHandler()));
routes.MapRoute(
name: "Default",
url: "{controller}/{action}/{id}",
defaults: new { controller = "Home", action
= "Index", id = UrlParameter.Optional }
);
}
}
}
5: Now ,there is no need to create Custom Action Method inside
Home/Demo because HttpHandler will get invoked before the ActionResult gets
Executed.
Go To HomeController and Add Below Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace HttpHandler.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
public ActionResult Demo()
{
return View();
}
}
}
6: Run The Application then displ
a
No comments:
Post a Comment